What are Events in JavaScript?
Event handling in JavaScript allows developers to create dynamic and interactive web applications. An event occurs when a user or browser triggers an action, such as clicking a button, pressing a key, or loading a webpage. For instance, actions like clicking a button, pressing a key, or loading a webpage are all events. These events allow developers to create interactive and dynamic web applications by executing JavaScript code in response to user interactions.
Event handling in JavaScript enables developers to execute specific actions based on user interactions, making web applications more engaging.
Types of Events in JavaScript
1. Mouse Events
Mouse events occur due to user actions with the mouse, such as clicking or moving over an element.
Examples include:
- click: Triggered when the user clicks on an element.
- dblclick: Fired when an element is double-clicked.
- mouseover: Occurs when the mouse pointer enters an element.
- mouseout: Triggered when the pointer leaves an element.
- mousemove: Fired when the mouse pointer moves over an element.
- mousedown: Triggered when a mouse button is pressed.
- mouseup: Triggered when a mouse button is released.
2. Keyboard Events
Keyboard events handle user interactions via keyboard inputs.
Examples include:
- keydown: Triggered when a key is pressed down.
- keyup: Triggered when a key is released.
- keypress (deprecated): Used when a key is pressed but is no longer recommended due to inconsistencies.
3. Form Events
Form events are triggered during interactions with forms and their elements.
Examples include:
- submit: Occurs when a form is submitted.
- reset: Triggered when a form is reset.
- focus: Fired when an element gains focus.
- blur: Fired when an element loses focus.
4. Document Events
Document events are triggered by changes or interactions with the document.
Examples include:
- load: Fired when the document or a resource is fully loaded.
- unload: Triggered when a page or resource is unloaded.
- scroll: Occurs when the document is scrolled.
- resize: Fired when the browser window is resized.
5. Window Events
Window events involve interactions or changes to the browser window.
Examples include:
- load: Triggered when all resources in the window are loaded.
- unload: Occurs when the window is about to close.
- scroll: Fired when the window is scrolled.
- resize: Triggered when the window is resized.
- focus: Occurs when the window gains focus.
- blur: Triggered when the window loses focus.
Event Handling Methods in JavaScript
JavaScript provides multiple ways to handle events effectively.
1. Inline Event Handling
Here, the event handler is added directly within the HTML element using an on attribute.
Example:
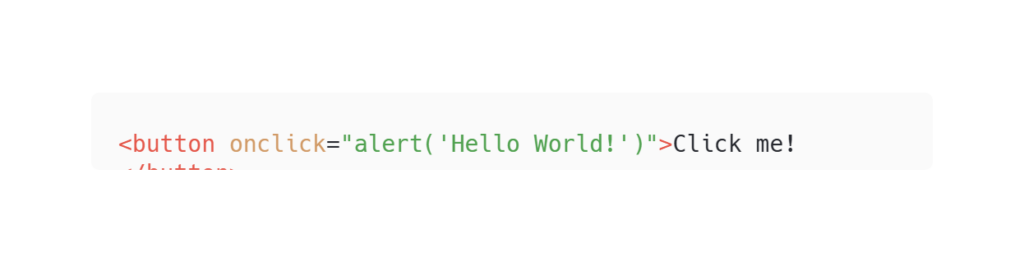
2. Traditional Event Handling
This method assigns the event handler directly to the element’s property in JavaScript.
Example:
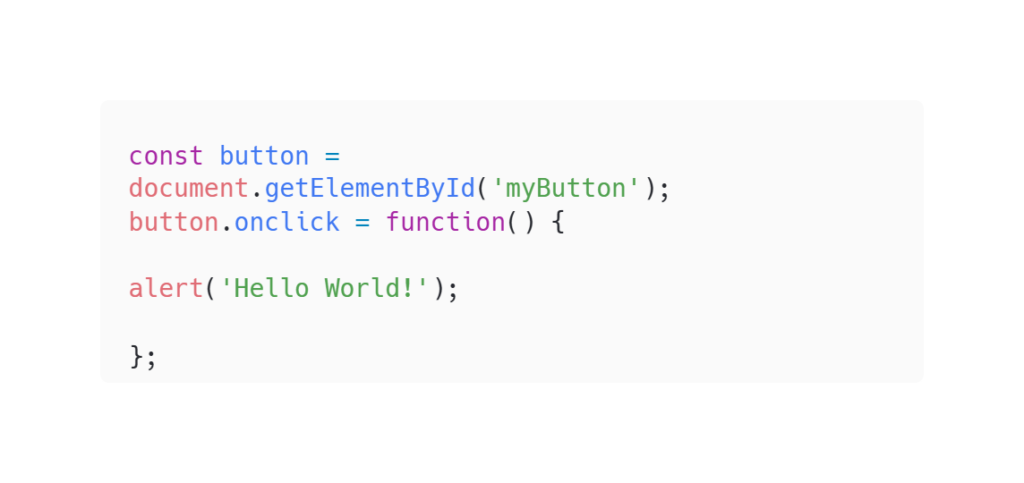
3. Modern Event Handling (Using addEventListener)
This method is the recommended approach because it allows multiple handlers for the same event and supports advanced options like event capturing.
Example:
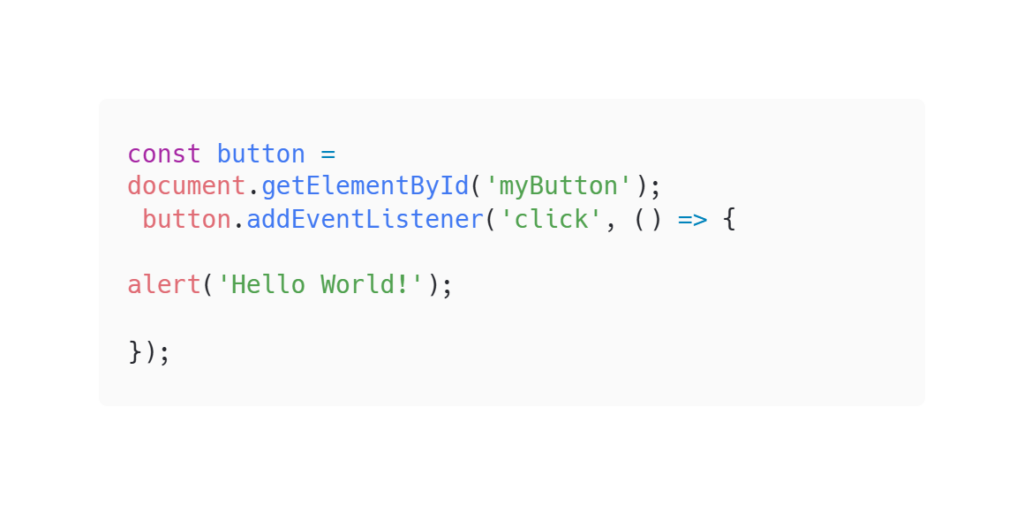
Event Object in JavaScript
The Event Object is automatically passed to the event handler function and contains details about the event.
Common Properties and Methods of the Event Object
- type: Indicates the type of event (e.g., click, submit).
- target: Refers to the element that triggered the event.
- currentTarget: Refers to the element currently handling the event.
- preventDefault(): Prevents the default browser behavior (e.g., stops form submission).
- stopPropagation(): Stops the event from propagating to other elements in the DOM.
Example:
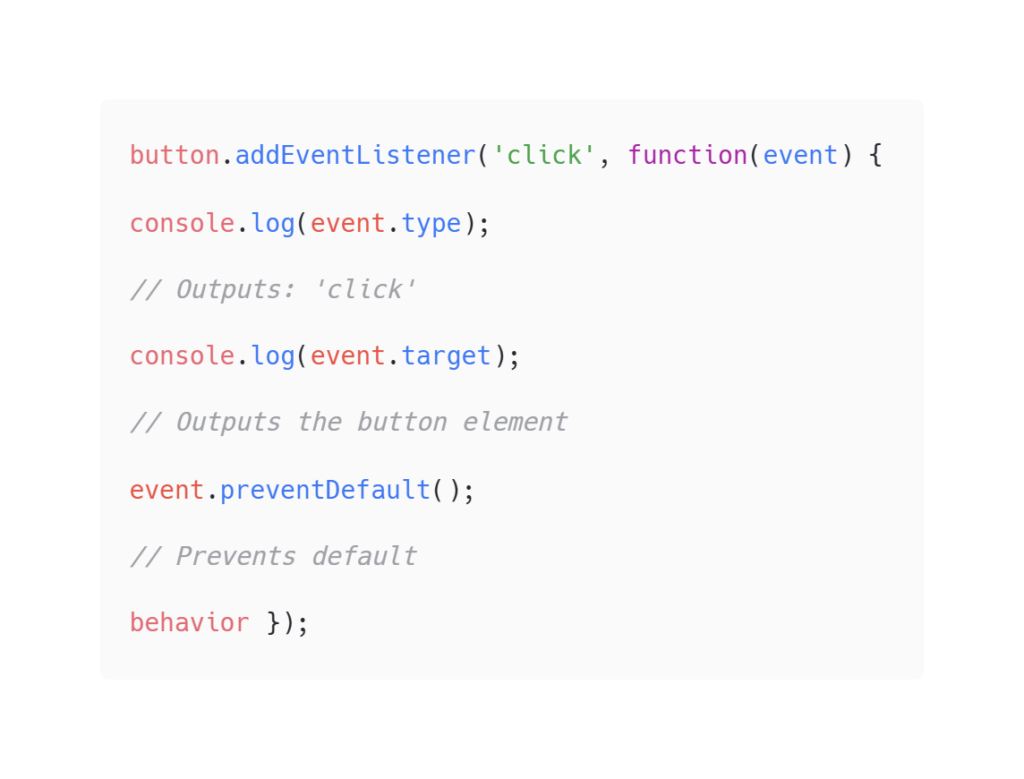
Event Delegation in JavaScript
Event delegation is a technique where a single event handler is added to a parent element to handle events on its child elements. This approach is particularly useful when working with dynamically generated elements.
Example:
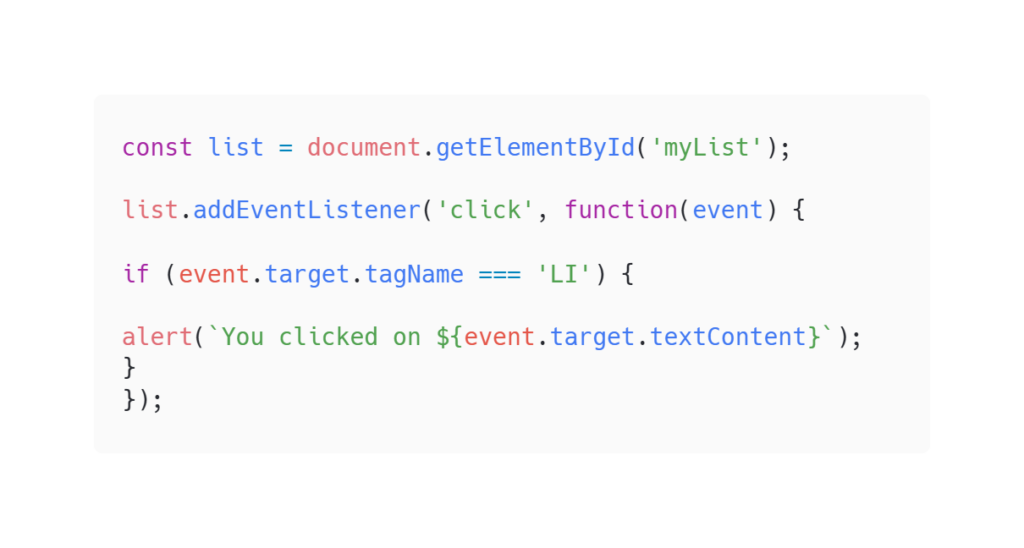
In this example, even if new <li> elements are added dynamically, the single event listener on the <ul> parent will manage the events.
Event Propagation: Bubbling and Capturing
When an event is triggered, it follows a two-phase path through the DOM:
- Capturing Phase (Event Capturing): The event starts from the root element (<html>) and travels down to the target element.
- Bubbling Phase (Event Bubbling): After reaching the target element, the event propagates back up to the root.
Example:
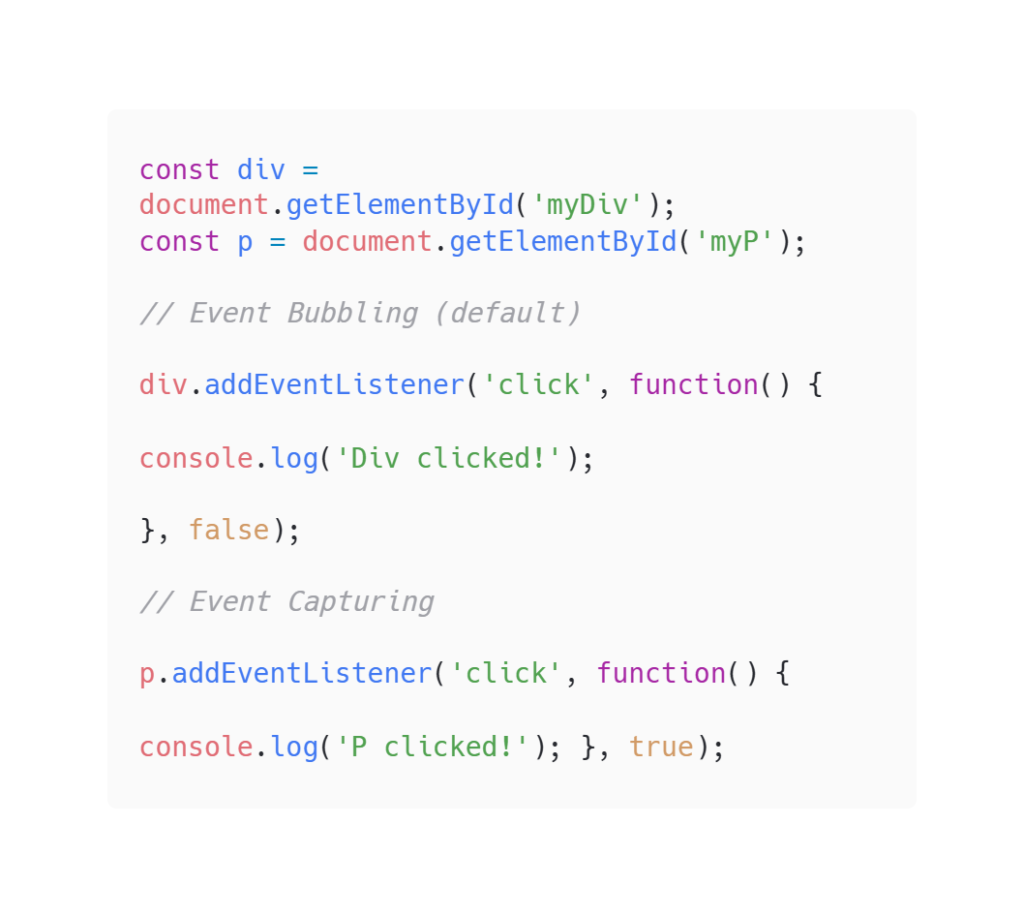
If the <p> element is clicked:
- During capturing, “P clicked!” is logged first.
- During bubbling, “Div clicked!” is logged next.
For a detailed guide on Event Handling in JavaScript, visit our website DesignWithRehana. Also, check out our YouTube videos for a hands-on demonstration of JavaScript events in action!