Ever opened a massive HTML file and felt lost in the chaos? Organizing large HTML documents properly is crucial because a poorly structured file can make updates a nightmare, slow down development, and hurt SEO. The good news? A well-organized HTML file isn’t just easier to manage—it also improves accessibility, readability, and performance.
If you want to learn HTML in detail you can checkout FREE HTML course also.
Let’s dive into the best practices for keeping your HTML clean, structured, and maintainable. I’ll also include real-world examples to make things crystal clear.
1. Use Clear, Meaningful Naming Conventions
If you’ve ever inherited a project full of cryptic class names like .box1 or .xyz, you know how frustrating it can be. Choosing clear, self-explanatory names makes your code easier to read and maintain.
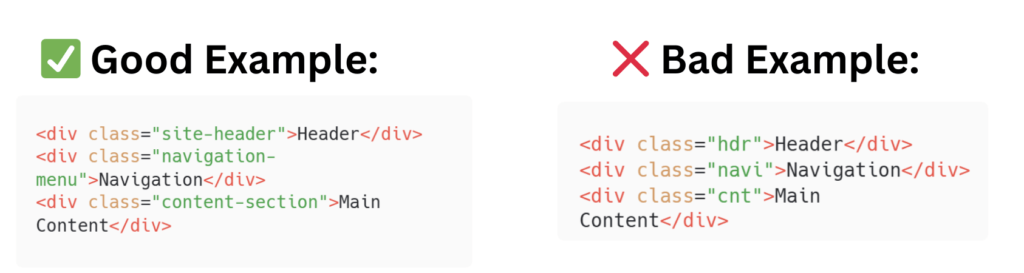
Why It Matters:
- Good: Descriptive names help you (and others) understand the code instantly.
- Bad: Vague abbreviations cause confusion and make debugging painful.
2. Use Semantic HTML for Better SEO & Accessibility
Overusing <div> tags instead of semantic elements is a common mistake. Semantic HTML helps search engines, screen readers, and developers understand your page structure.
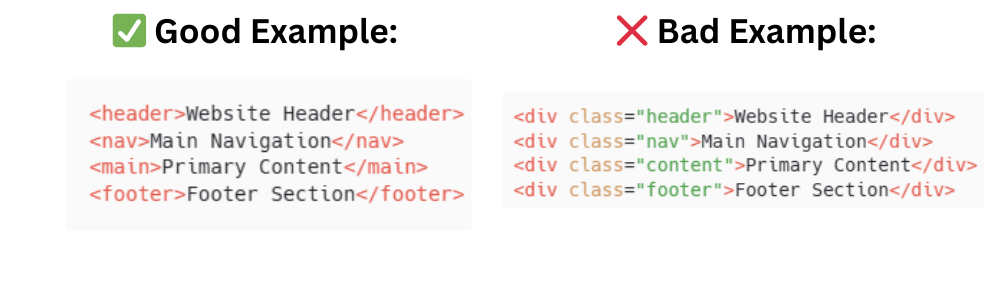
Why It Matters:
- Good: Enhances SEO and improves accessibility.
- Bad: Generic <div> tags don’t convey meaning, making it harder for search engines to rank your site.
3. Keep Your Code Readable with Proper Line Breaks
A single, unbroken line of HTML is hard to read and debug. Breaking it into multiple lines improves clarity and makes troubleshooting easier.

Why It Matters:
- Good: Easier to scan, edit, and debug.
- Bad: One-liner code is messy and frustrating to maintain.
4. Use Proper Indentation
Indentation visually represents the hierarchy of your elements, making it easier to understand their relationships.
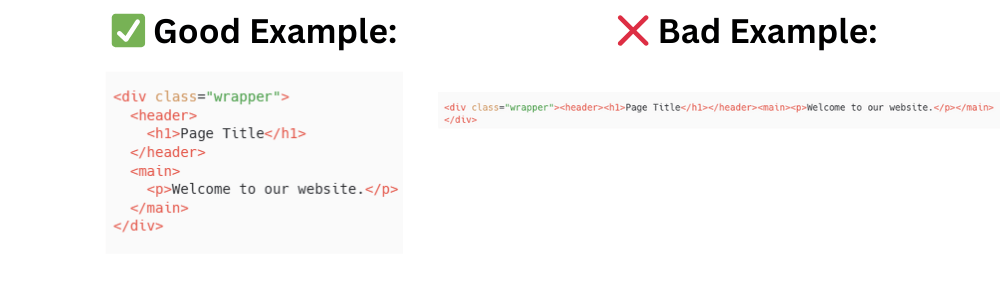
Why It Matters:
- Good: Maintains a logical structure that’s easy to follow.
- Bad: Without indentation, finding errors in large files is a hassle.
5. Add Comments to Improve Code Understanding
Whether you’re working alone or with a team, comments help document your code, making future updates easier.
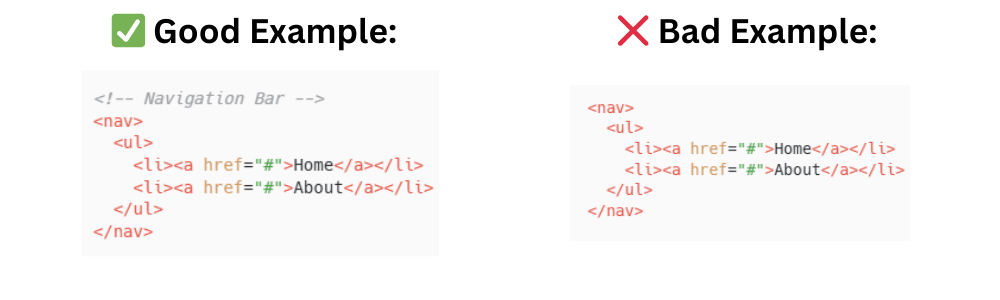
Why It Matters:
- Good: Comments provide context for complex sections.
- Bad: Without them, you may waste time figuring out what each section does.
6. Keep Your Code Modular
Instead of cramming everything into a single file, break your HTML into reusable sections like Header, Navigation, and Footer.
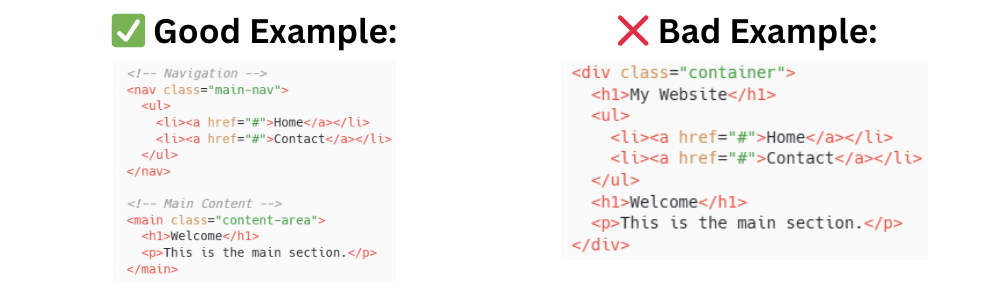
Why It Matters:
- Good: Makes your code reusable, scalable, and maintainable.
- Bad: Mixing everything in one <div> creates a mess.
7. Avoid Inline Styles – Use External Stylesheets
Embedding styles directly into HTML makes updates difficult. Instead, use a CSS file or a preprocessor like SASS.
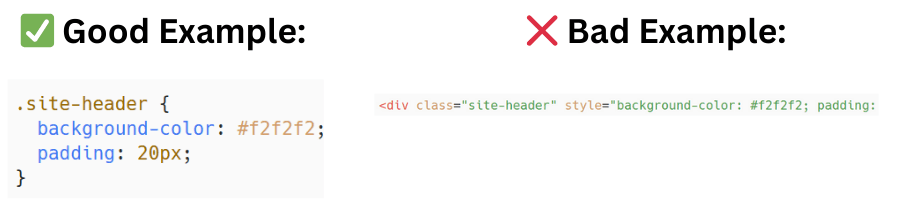
Why It Matters:
- Good: Keeps styles modular and maintainable.
- Bad: Inline styles clutter HTML and are hard to manage.
8. Use an HTML Linter for Error-Free Code
Linting tools like HTMLHint or W3C Validator catch mistakes before they become problems.
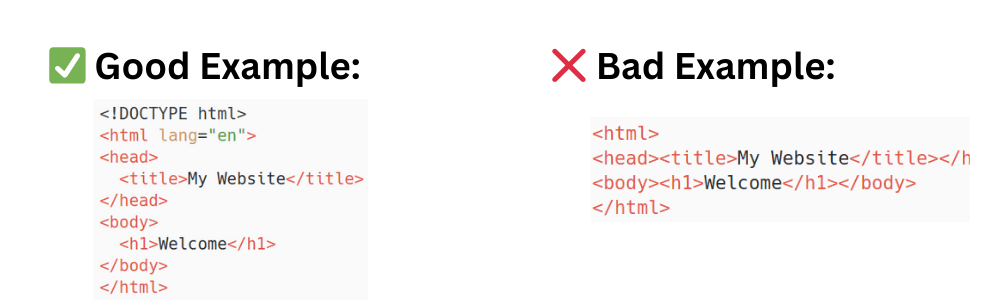
Why It Matters:
- Good: Helps you catch errors early.
- Bad: Poorly structured HTML can break layouts.
9. Maintain a Well-Organized File Structure
A well-organized project is easier to navigate and debug.
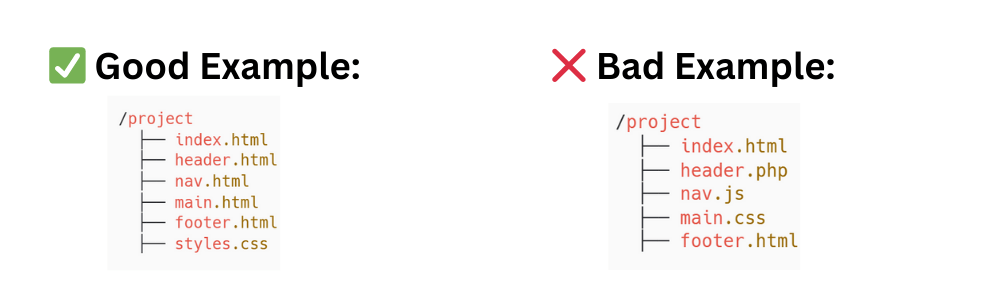
Why It Matters:
- Good: Keeps related files together, improving organization.
- Bad: A disorganized structure slows development.
Final Thoughts
Following these best practices will make organizing large HTML documents easier, resulting in cleaner, more readable, and SEO-friendly code. Whether you’re building a small site or a large-scale project, well-structured code saves time and effort in the long run.Check out my HTML course on YouTube to learn more!