Asynchronous JavaScript
Asynchronous JavaScript is a programming approach that lets your code perform multiple tasks simultaneously without waiting for one task to finish before starting another. This is particularly helpful for handling operations that take time, such as fetching data from a server, accessing a database, or reading files. By preventing the main thread from getting stuck, it keeps applications responsive and improves performance—making it an essential part of web development.
Callbacks
A callback is a function passed as an argument to another function, which is executed once the initial task is complete. Callbacks are a straightforward way to handle asynchronous tasks in JavaScript.
Example:
Javascript code
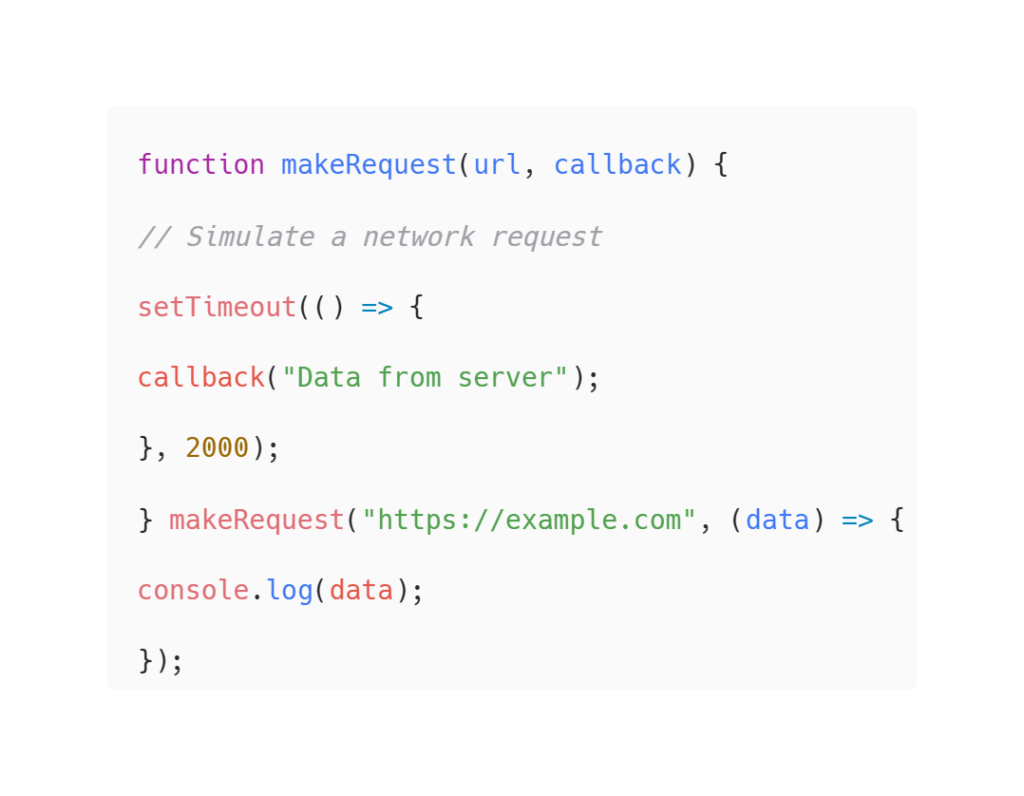
Explanation:
- The makeRequest function takes two arguments: a URL and a callback function.
- It simulates a network request using setTimeout. After a delay of 2 seconds, it calls the callback function and passes the response data (“Data from server”) to it.
- The callback handles the result of the asynchronous operation.
While functional, excessive use of callbacks can lead to nested, hard-to-read code, often referred to as “callback hell.”
Promises
Promises provide a cleaner and more manageable way to handle asynchronous operations. A promise represents a value that might not be available yet but will either resolve (complete successfully) or reject (fail) at some point in the future.
Example:Javascript code
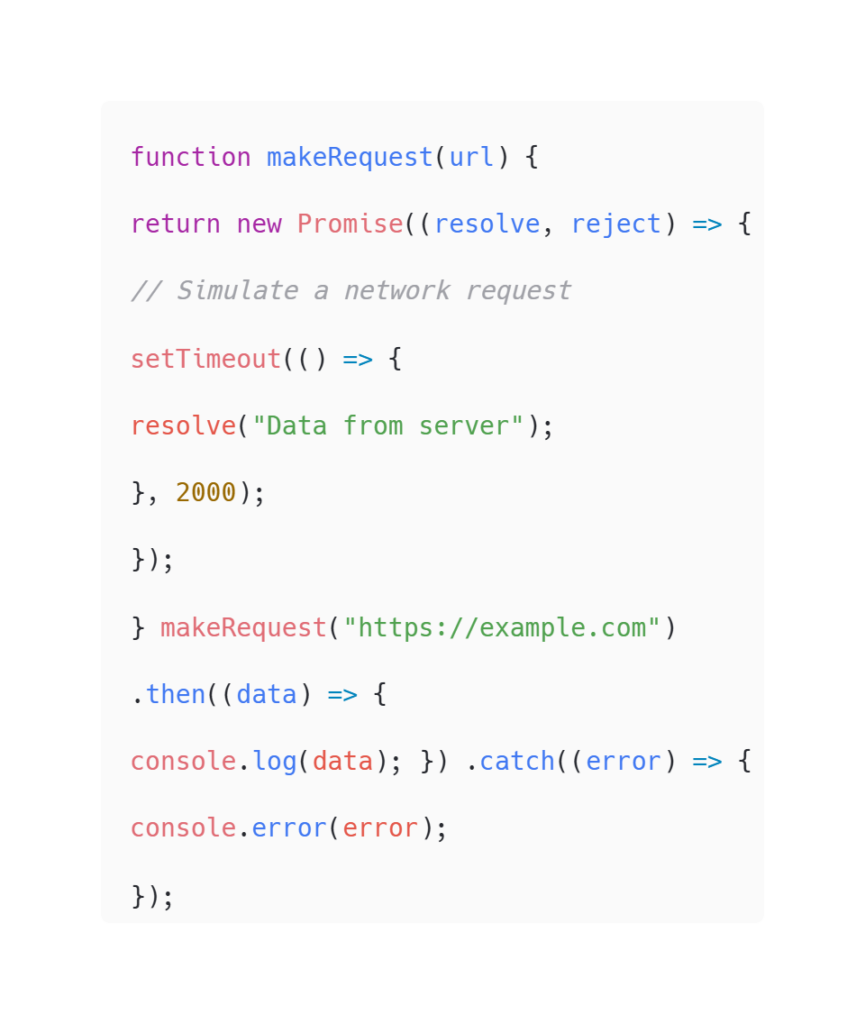
Explanation:
- The makeRequest function returns a promise.
- Inside the promise, the resolve function is called when the operation is successful, passing the response data (“Data from server”).
- The .then() method processes the resolved promise, while .catch() handles errors if the promise is rejected.
Promises simplify the structure of asynchronous code compared to callbacks, making it more readable.
Async/Await
Async/await is a syntax built on top of promises that allows you to write asynchronous code in a way that looks and behaves like synchronous code. It simplifies error handling and improves readability.
Example:
Javascript code
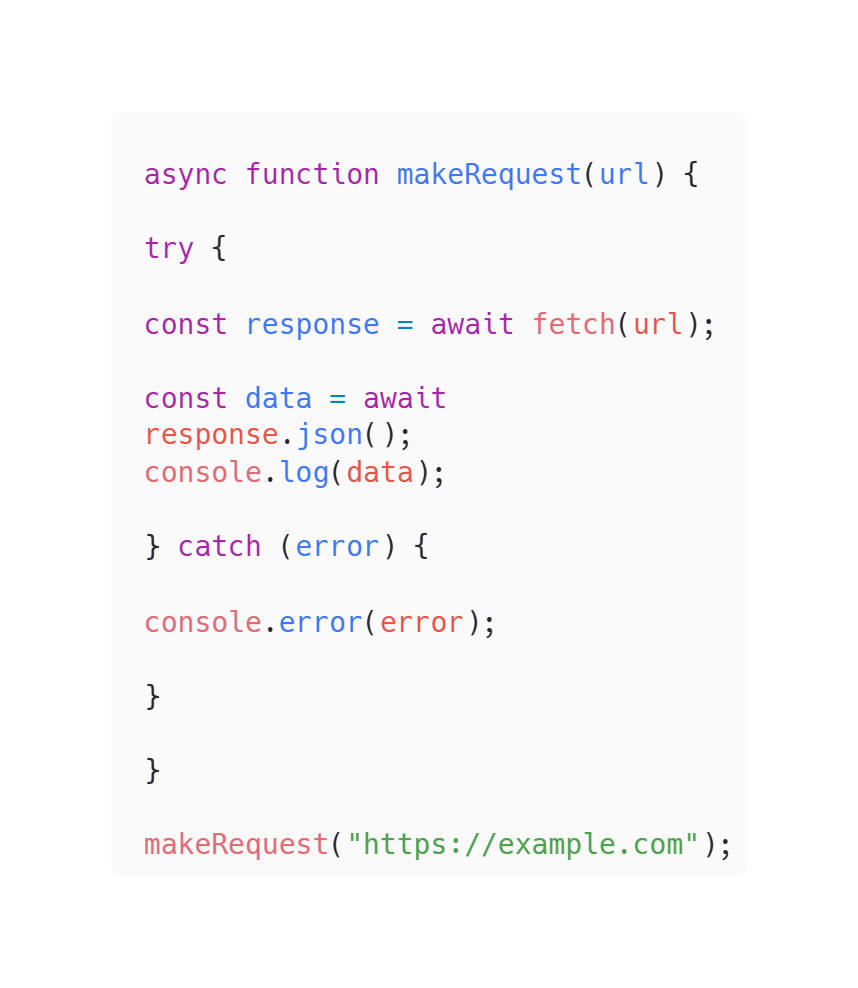
Explanation:
- The async keyword declares an asynchronous function.
- The await keyword pauses the execution of the function until the promise resolves, ensuring the result is available before continuing.
- The try/catch block captures errors, such as network failures or invalid responses, and handles them gracefully.
Async/await enhances readability and is often preferred for writing asynchronous code in modern JavaScript.
Real-World Example
In real-world applications, asynchronous JavaScript is widely used for tasks like fetching data from APIs. Here’s a practical example:
Javascript code
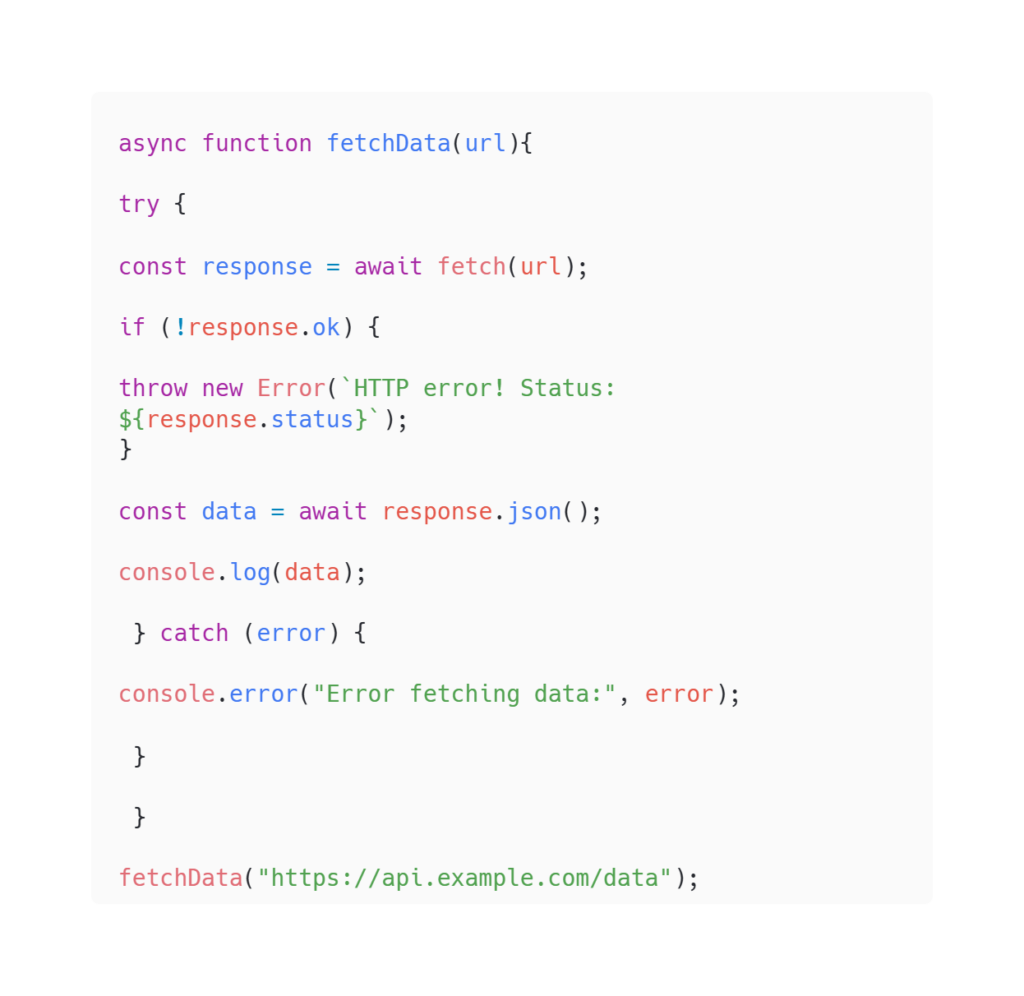
Explanation:
- The fetchData function uses the fetch API to make a network request.
- The await keyword ensures the function waits for the API response before proceeding.
- If the response is successful, the data is logged; otherwise, an error is thrown and handled in the catch block
Summary
Asynchronous JavaScript helps manage tasks that take time to complete without freezing the application. Here’s a quick comparison of the approaches:
Callbacks: Useful for basic asynchronous operations but can lead to complex, nested code structures (“callback hell”).
Promises: Simplify asynchronous workflows, making the code cleaner and easier to follow.
Async/Await: Further improves readability by allowing asynchronous code to be written in a synchronous style, with straightforward error handling.
To learn more about JavaScript and web development, check out my detailed guide on asynchronous JavaScript on my website DesignWithRehana . Also, don’t forget to subscribe to my YouTube channel for more tutorials and coding insights!